05 May 2020
目前 .NET Core 範本提供的是 Angular 8, 但是想使用新的版本建立專案所以研究了一下
-
使用 ASP.NET Core with Angular
範本建立專案.
dotnet new angular
-
移除專案內的 ClientApp
資料夾
-
使用 Angular CLI 建立新的 Angular 專案
ng new ClientApp --skipGit --skipInstall
-
修改 angular.json
內的輸出路徑, 讓 .NET Core 在 publish 或 debug 時可以找到 Angular 前端檔案
{
"options": {
"outputPath": "dist"
}
}
-
如果 .NET Core 在 debug 時一直無法正確開啟前端專案, 則將 package.json
內的 ng server
修改成 ng serve --verbose
{
"scripts": {
"start": "ng serve --verbose"
}
}
Publish
dotnet publish -c release
修改 html base tag 的 href 屬性
如果網站不是放在網站根目錄, 需要修改 package.json
裡的 build
指令, 或是發行後手動修改 index.html
裡的 base
HTML tag.
{
"scripts": {
"build": "ng build --base-href=/YourBasePath/"
}
}
19 Apr 2020
首先先勾選選項啟動 k8s
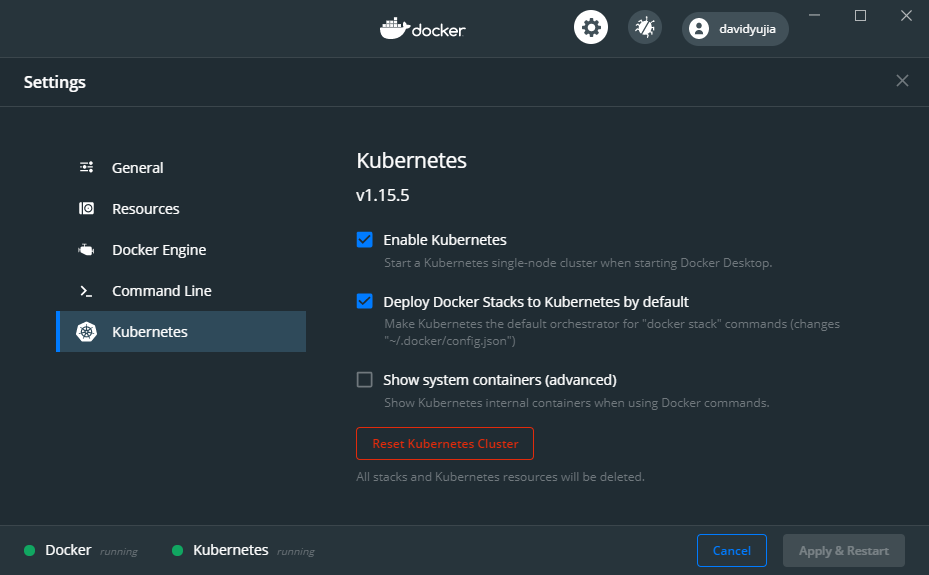
再來從 kubernetes dashboard releases 選擇 k8s dashboard 版本,這裡使用 v1.10.1
打開 powershell 輸入
kubectl apply -f https://raw.githubusercontent.com/kubernetes/dashboard/v1.10.1/src/deploy/recommended/kubernetes-dashboard.yaml
執行 kubectl proxy
, 接著 command-line 上會顯示
Starting to serve on 127.0.0.1:8001
接著我們可以打開 web browser 連到 http://localhost:8001/api/v1/namespaces/kube-system/services/https:kubernetes-dashboard:/proxy/ 則會出現登入畫面, 可以使用 kubeconfig 或 token 登入
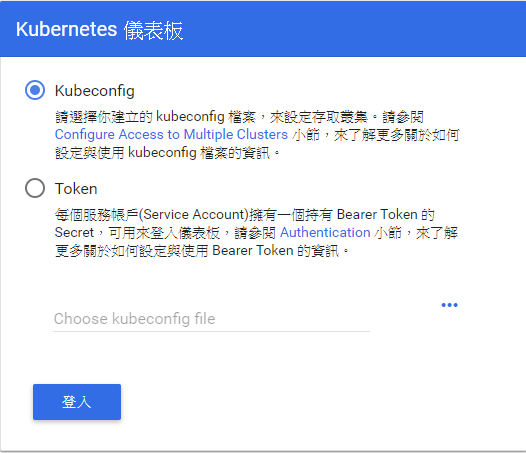
直接輸入下面指令直接取得 token
kubectl -n kube-system describe secret default
config 可以在 %USERPROFILE%\.kube
下找到, 不過試了幾個方式還沒成功, 所以這邊直接複製 token 登入
之後就可以看到 dashboard 的畫面了
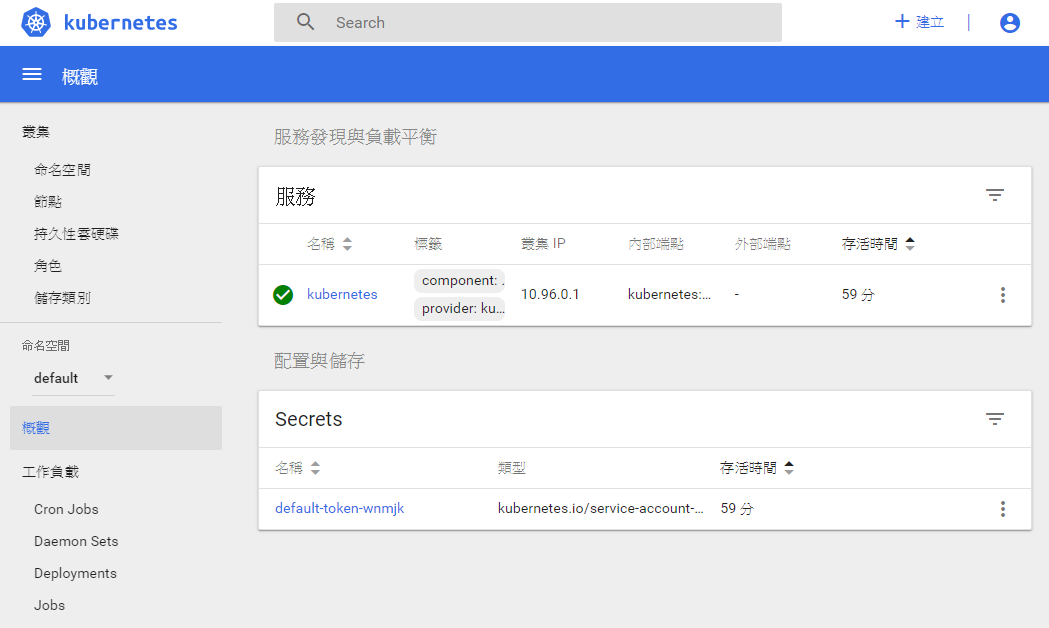
23 Mar 2020
安裝套件
這邊使用版本 3.1.2
dotnet add package Microsoft.Extensions.Configuration.Json --version 3.1.2
在 .cs
var config = new ConfigurationBuilder()
.AddJsonFile("appSettings.json", optional: true)
.AddJsonFile($"appSettings.{Environment.GetEnvironmentVariable("ASPNETCORE_ENVIRONMENT")}.json", optional: true)
.Build();
取得 connect string
var connectionString = config.GetConnectionString("DefaultConnection");
取得 value
var logPath = config.GetSection("LogPath").Value;
使用 Model 包裝
var mailSetting = config.GetSection("MailSettings").Get<MailSettingModel>();
23 Mar 2020
安裝 EF 工具
dotnet tool install dotnet-ef -g
將 Microsoft.EntityFrameworkCore.Design 加入至專案
5.0.0
目前是 preview 版本, 這邊安裝 3.1.2
dotnet add package Microsoft.EntityFrameworkCore.Design --version 3.1.2
從 DB 產生 Models
根據不同的 DB 安裝不同的 Provider, 例如: Microsoft.EntityFrameworkCore.SqlServer
.
dotnet ef dbcontext scaffold "Server=(localdb)\MSSQLLocalDB;Database=ContosoUniversity;Trusted_Connection=True" Microsoft.EntityFrameworkCore.SqlServer -o Models -f -c SqlDbContext
此指令會使用 Microsoft.EntityFrameworkCore.SqlServer
將 Model 輸出在 Models
資料夾, 並把 DbContext 命名為 SqlDbContext
.
使用 Nuget 套件管理員可以使用以下指令
Scaffold-DbContext "Server=(localdb)\MSSQLLocalDB;Database=ContosoUniversity;Trusted_Connection=True" Microsoft.EntityFrameworkCore.SqlServer -OutputDir Models -f -c SqlDbContext
Providers
以下是目前在專案使用過的 Providers
DB |
Provider |
|
MSSQL |
Microsoft.EntityFrameworkCore.SqlServer |
Link |
PostgreSQL |
Npgsql.EntityFrameworkCore.PostgreSQL |
Link |
使用 “user-secrets” 儲存連線字串
首先先初始化 user-secrets
dotnet user-secrets init
再將連線字串儲存至 user-secrets
dotnet user-secrets set ConnectionStrings.DefaultConnection "Server=(localdb)\MSSQLLocalDB;Database=ContosoUniversity;Trusted_Connection=True"
最後將連線字串修改成 Name=DefaultConnection
dotnet ef dbcontext scaffold Name=DefaultConnection Microsoft.EntityFrameworkCore.SqlServer
在 Console 中使用 DbContext
var option = new DbContextOptionsBuilder<SqlTaikooContext>().UseSqlServer(connectionString).Options;
var context = new SqlDbContext(option);
參考資料:
https://docs.microsoft.com/zh-tw/ef/core/managing-schemas/scaffolding